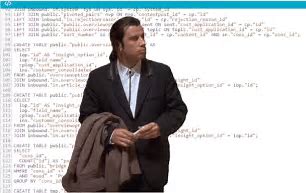
Let’s embark on a journey into the enchanting realm of SQLAlchemy, where Object-Relational Mapping (ORM) adds a layer of magic to database interactions. We’ll unravel the secrets of configuring Mapped Classes, the wizards behind the scenes.
Understanding ORM Mapped Classes
In SQLAlchemy, Mapped Classes serve as the bridge between the world of objects in your code and the rows in your database tables. To make this magic happen, let’s explore the essential configurations for Mapped Classes.
Creating a Mapped Class
from sqlalchemy import Column, Integer, String
from sqlalchemy.orm import declarative_base
Base = declarative_base()
class YourModel(Base):
__tablename__ = 'your_table_name'
id = Column(Integer, primary_key=True)
name = Column(String)
age = Column(Integer)
Here, YourModel becomes the hero of your database story, with attributes corresponding to the columns in your table.
Defining Relationships
The beauty of SQLAlchemy lies in its ability to represent relationships between tables effortlessly. Let’s say you have another model, AnotherModel
, and you want to establish a relationship between them.
from sqlalchemy import ForeignKey
from sqlalchemy.orm import relationship
class AnotherModel(Base):
__tablename__ = 'another_table'
id = Column(Integer, primary_key=True)
something_else = Column(String)
your_model_id = Column(Integer, ForeignKey('your_model.id'))
your_model = relationship('YourModel', back_populates='another_models')
Here, the relationship
attribute creates a connection between the two models, enabling you to navigate the relationships seamlessly.
Additional Configuration Options
- Customizing Table Names
If your class name and table name aren’t a perfect match, you can specify it using__tablename__
:
your_model = relationship('YourModel', back_populates='another_models', uselist=False)
2. Handling Relationships
Use the relationship
attribute to define relationships, specifying details like back-references and joining conditions.
your_model = relationship('YourModel', back_populates='another_models', uselist=False)
3. Indexing Columns
Improve query performance by adding indexes to columns:
name = Column(String, index=True)
Bringing It All Together
from sqlalchemy import Column, Integer, String, ForeignKey
from sqlalchemy.orm import relationship
from sqlalchemy.ext.declarative import declarative_base
Base = declarative_base()
class YourModel(Base):
__tablename__ = 'your_table_name'
id = Column(Integer, primary_key=True)
name = Column(String)
age = Column(Integer)
another_models = relationship('AnotherModel', back_populates='your_model')
class AnotherModel(Base):
__tablename__ = 'another_table'
id = Column(Integer, primary_key=True)
something_else = Column(String)
your_model_id = Column(Integer, ForeignKey('your_model.id'))
your_model = relationship('YourModel', back_populates='another_models')
There you have it! With the power of ORM Mapped Class Configuration in SQLAlchemy, you’re ready to weave complex relationships and transform your database interactions into a magical experience. Happy coding! 🚀💻