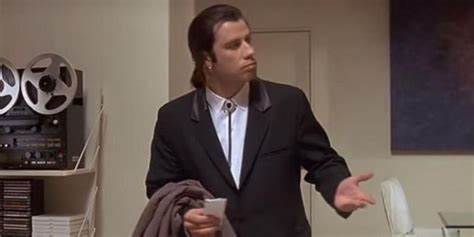
Object-Relational Mapping (ORM) is a technique that lets you query and manipulate data from a database using an object-oriented paradigm. When talking about ORM, most people are referring to a library that implements the Object-Relational Mapping technique, hence the phrase “an ORM”.
An ORM library is a completely ordinary library written in your language of choice that encapsulates the code needed to manipulate the data, so you don’t use SQL anymore; you interact directly with an object in the same language you’re using.
For example, here is a completely imaginary case with a pseudo language:
You have a book class, you want to retrieve all the books of which the author is “biko”. Manually, you would do something like that:
book_list = new List();
sql = "SELECT book FROM library WHERE author = 'biko'";
data = query(sql); // I over simplify ...
while (row = data.next())
{
book = new Book();
book.setAuthor(row.get('author');
book_list.add(book);
}
SQLAlchemy
SQLAlchemy is the Python SQL toolkit and Object Relational Mapper that gives application developers the full power and flexibility of SQL.
It provides a full suite of well known enterprise-level persistence patterns, designed for efficient and high-performing database access, adapted into a simple and Pythonic domain language.
Managing the state of your data is crucial for robust applications, and SQLAlchemy ORM offers a powerful toolkit for handling this with elegance and efficiency. 💼
In SQLAlchemy, the ORM layer helps us bridge the gap between the relational database and our Python code, making it seamless to work with database records as Python objects. 🐍
Key points on state management with SQLAlchemy ORM:
- Identity Map Pattern: SQLAlchemy keeps track of objects in a unit of work using the Identity Map pattern. This ensures that each database row is represented by a single Python object within a session.
- Session State: The
Session
in SQLAlchemy acts as a staging area for all changes before committing them to the database. Understanding and managing session states is vital for a smooth workflow. - Dirty Tracking: SQLAlchemy automatically tracks changes to objects and only updates the fields that have been modified. This minimizes unnecessary database updates, optimizing performance.
- Unit of Work Pattern: SQLAlchemy employs the Unit of Work pattern to coordinate changes and transactions, making it easier to manage complex operations involving multiple objects.
- Flushing and Committing: Explicitly flushing the session synchronizes changes with the database, and committing finalizes the transaction. Understanding when to flush and commit is essential for data integrity.
- Events and Hooks: SQLAlchemy provides a rich set of events and hooks, allowing customization of state management behaviors to suit specific application needs.
Assume you have a User
model representing users in a database:
from sqlalchemy import create_engine, Column, Integer, String, Sequence
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
# Define the User model
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
id = Column(Integer, Sequence('user_id_seq'), primary_key=True)
name = Column(String(50))
age = Column(Integer)
# Create an SQLite in-memory database and bind the engine
engine = create_engine('sqlite:///:memory:')
# Create the 'users' table in the database
Base.metadata.create_all(engine)
# Create a Session class to interact with the database
Session = sessionmaker(bind=engine)
session = Session()
Now, let’s explore state management:
# Create a new user and add it to the session
new_user = User(name='John Doe', age=25)
session.add(new_user)
# At this point, the user is in the 'Pending' state, not yet committed to the database
# Query the user (this will trigger a flush to the database)
queried_user = session.query(User).filter_by(name='John Doe').first()
# The queried_user is now in the 'Persistent' state, representing a database record
# Modify the user's age
queried_user.age = 26
# At this point, SQLAlchemy has marked the user as 'Dirty' because it has been modified
# Commit the changes to the database
session.commit()
# Now, the changes are saved to the database, and the user is in the 'Committed' state
# The session is now in a 'Clean' state, and the user is no longer marked as 'Dirty'
In this example:
- Pending: When a new user is added to the session, it is in a pending state.
- Persistent: After querying a user from the database, it becomes persistent.
- Dirty: If you modify a persistent object, it becomes dirty.
- Committed: After committing changes, the object is in a committed state.
- Clean: The session is clean after committing, and the object is no longer dirty.
Understanding these states helps you manage your data effectively when working with SQLAlchemy ORM. Feel free to experiment and build upon this example!